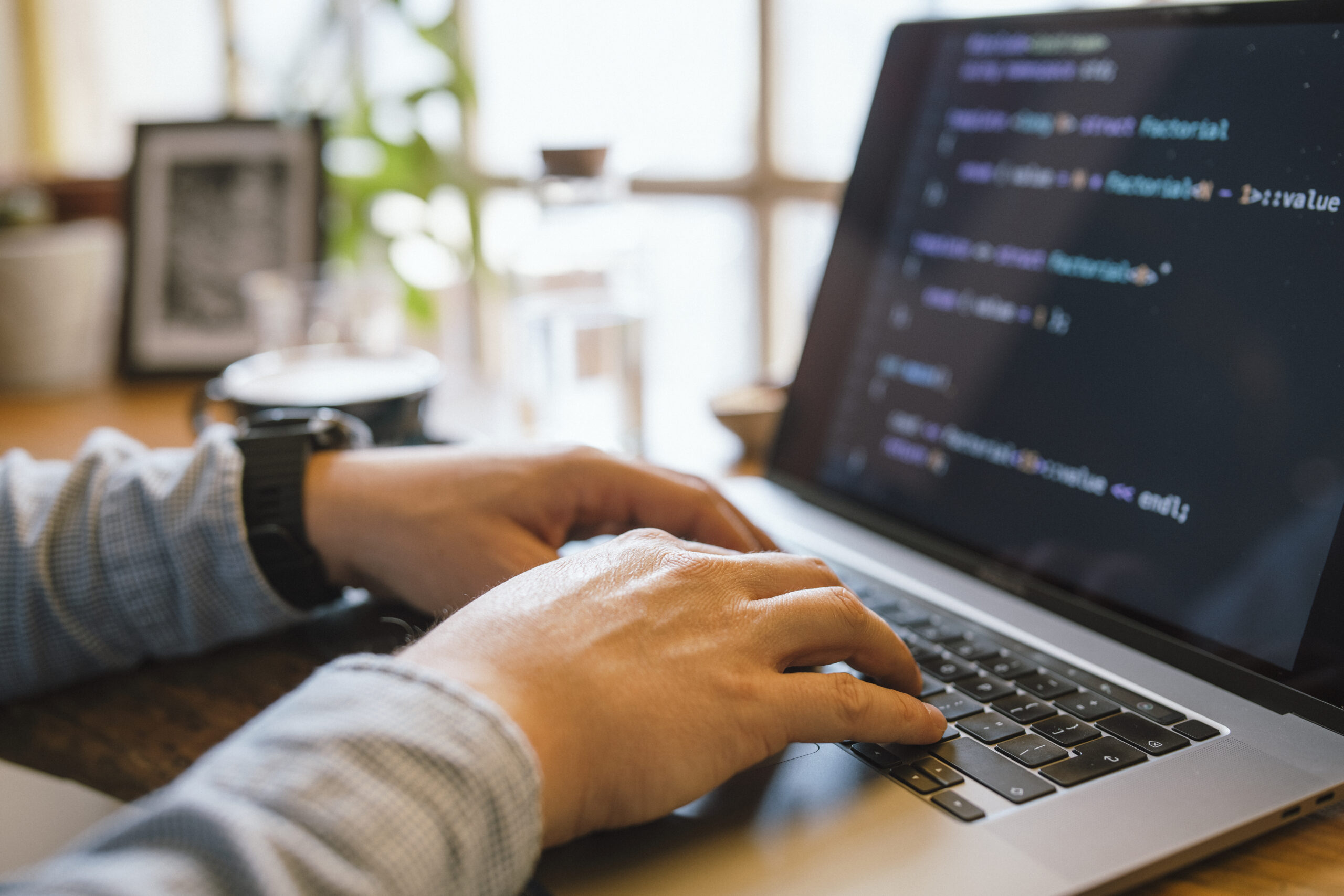
Debugging is Probably the most vital — yet usually neglected — competencies in a developer’s toolkit. It isn't pretty much correcting damaged code; it’s about understanding how and why issues go Improper, and Finding out to think methodically to solve problems efficiently. Regardless of whether you're a newbie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and considerably transform your efficiency. Here are quite a few procedures that will help builders degree up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
One of many quickest means builders can elevate their debugging competencies is by mastering the instruments they use every single day. Although creating code is one part of enhancement, knowing ways to interact with it efficiently throughout execution is Similarly crucial. Modern enhancement environments appear equipped with highly effective debugging capabilities — but many developers only scratch the floor of what these resources can perform.
Get, for instance, an Built-in Growth Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, phase via code line by line, and perhaps modify code within the fly. When used accurately, they let you observe specifically how your code behaves during execution, that's a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, check out serious-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip annoying UI challenges into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate in excess of functioning processes and memory management. Finding out these instruments may have a steeper Understanding curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with version Handle units like Git to know code background, uncover the precise minute bugs were being released, and isolate problematic adjustments.
In the long run, mastering your instruments usually means likely further than default configurations and shortcuts — it’s about developing an intimate understanding of your growth natural environment to make sure that when challenges crop up, you’re not shed at midnight. The better you understand your resources, the more time you are able to invest fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most vital — and often overlooked — steps in helpful debugging is reproducing the situation. In advance of jumping in the code or generating guesses, developers need to produce a regular surroundings or scenario in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a game of prospect, typically resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Ask thoughts like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or mistake messages? The greater depth you have, the much easier it gets to be to isolate the precise situations less than which the bug takes place.
After you’ve gathered adequate information and facts, try and recreate the problem in your neighborhood surroundings. This may imply inputting a similar info, simulating identical user interactions, or mimicking process states. If The problem seems intermittently, think about producing automatic exams that replicate the sting conditions or state transitions involved. These exams not simply help expose the challenge but will also stop regressions Sooner or later.
At times, The problem may very well be atmosphere-distinct — it'd happen only on specific running methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a mindset. It demands persistence, observation, plus a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. By using a reproducible state of affairs, you can use your debugging resources much more efficiently, check prospective fixes securely, and talk additional Plainly with the staff or end users. It turns an summary grievance into a concrete challenge — and that’s exactly where developers prosper.
Examine and Have an understanding of the Mistake Messages
Error messages are frequently the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really learn to take care of mistake messages as direct communications from the procedure. They generally inform you precisely what occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Start out by looking through the message cautiously As well as in entire. Numerous builders, particularly when under time force, glance at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the accurate root trigger. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them initially.
Break the mistake down into components. Is it a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can considerably speed up your debugging method.
Some mistakes are obscure or generic, As well as in those circumstances, it’s very important to examine the context during which the mistake occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These typically precede larger sized problems and provide hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, helping you pinpoint problems more rapidly, lower debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most highly effective applications inside of a developer’s debugging toolkit. When made use of effectively, it provides real-time insights into how an application behaves, helping you comprehend what’s happening under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts off with recognizing what to log and at what level. Typical logging levels include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic info for the duration of growth, Data for common occasions (like successful get started-ups), Alert for likely concerns that don’t split the application, Mistake for genuine difficulties, and FATAL in the event the process can’t proceed.
Steer clear of flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your system. Center on essential occasions, point out improvements, input/output values, and critical final decision points in the code.
Format your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a properly-assumed-out logging solution, you are able to decrease the time it's going to take to spot concerns, attain deeper visibility into your programs, and Enhance the Over-all maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's click here a type of investigation. To properly detect and resolve bugs, builders will have to approach the process like a detective fixing a thriller. This way of thinking allows break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indications of the problem: error messages, incorrect output, or overall performance problems. Much like a detective surveys a criminal offense scene, gather as much related details as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear picture of what’s happening.
Up coming, type hypotheses. Request by yourself: What could possibly be leading to this conduct? Have any variations a short while ago been designed towards the codebase? Has this issue happened in advance of beneath equivalent situations? The aim is always to narrow down options and discover likely culprits.
Then, exam your theories systematically. Try to recreate the condition in a very controlled ecosystem. If you suspect a selected operate or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the final results lead you nearer to the truth.
Pay near interest to tiny details. Bugs generally hide during the minimum envisioned spots—like a missing semicolon, an off-by-one error, or even a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely understanding it. Momentary fixes may cover the actual challenge, only for it to resurface later on.
Last of all, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for future challenges and assist Some others understand your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and turn out to be simpler at uncovering concealed problems in intricate units.
Create Exams
Composing checks is among the most effective methods to increase your debugging techniques and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety net that provides you self confidence when making modifications in your codebase. A properly-examined application is simpler to debug since it lets you pinpoint just wherever and when a challenge happens.
Get started with device checks, which center on unique capabilities or modules. These smaller, isolated assessments can promptly reveal no matter whether a particular piece of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to look, noticeably lessening enough time put in debugging. Unit tests are Primarily useful for catching regression bugs—challenges that reappear just after Earlier getting fixed.
Future, combine integration exams and end-to-close assessments into your workflow. These aid be certain that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with multiple parts or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a element correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing naturally qualified prospects to raised code construction and less bugs.
When debugging an issue, producing a failing test that reproduces the bug might be a robust first step. When the test fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you catch more bugs, more quickly and a lot more reliably.
Choose Breaks
When debugging a tough issue, it’s straightforward to become immersed in the challenge—observing your monitor for several hours, trying Answer right after Alternative. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your thoughts, minimize stress, and sometimes see The problem from a new viewpoint.
When you're also near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind results in being less efficient at trouble-resolving. A brief stroll, a coffee crack, or maybe switching to a unique activity for 10–quarter-hour can refresh your concentration. Quite a few developers report discovering the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the qualifications.
Breaks also support avoid burnout, especially all through extended debugging periods. Sitting down before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Strength along with a clearer mentality. You could possibly abruptly notice a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, a very good guideline is to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all around, stretch, or do anything unrelated to code. It may come to feel counterintuitive, Specifically less than restricted deadlines, but it really truly causes quicker and simpler debugging in the long run.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a smart approach. It presents your brain House to breathe, improves your point of view, and allows you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is much more than just a temporary setback—It really is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each one can educate you anything important if you make an effort to mirror and examine what went Erroneous.
Start by asking your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit tests, code reviews, or logging? The responses often expose blind spots in your workflow or comprehending and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be an outstanding practice. Retain a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. After some time, you’ll begin to see designs—recurring troubles or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've realized from a bug with all your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief compose-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. After all, several of the very best builders aren't those who write best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So subsequent time you squash a bug, take a instant to mirror—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Strengthening your debugging skills will take time, exercise, and tolerance — however the payoff is big. It will make you a more effective, self-confident, and able developer. The next time you're knee-deep in the mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become greater at Anything you do.